In this tutorial I will show you how to parse a hand-drawn hash game with Computer Vision techniques to determine who’s the winner (if any). See the example below:
Eye Tracking for Mouse Control in OpenCV
In this tutorial I will show you how you can control your mouse using only a simple webcam. Nothing fancy, super simple to implementate. Let’s get on!
Multithreaded K-Means in Java
Single-threaded algorithms are fine and intuitive. There’s an single execution flow running our program in the exact order we specified it. However, modern CPUs have multiple cores sharing the same memory space, meaning that many instructions can be executed in the same clock cycle. Having an single execution flow would be a wasteful use of the resources we have available. That’s where multithread applications come in: It allows the use of true parallelism.
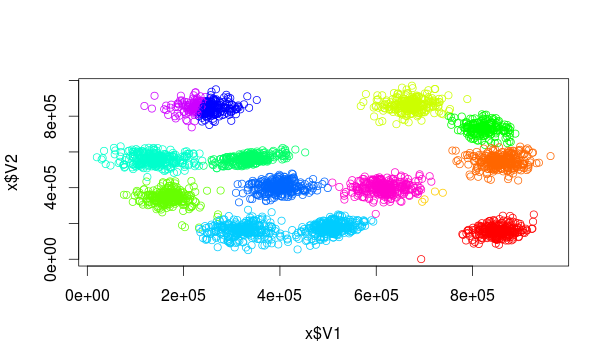
Mixing Assembly and C
In many applications, mixing Assembly and C is routine (pun intended). There are many reasons for it, but, in general, you want to use Assembly when you want to deal with the hardware directly or perform a task with maximum speed and minimum use of resources, while you use C to perform some high level stuffs that don’t attend the former requirements. In either case, you’ll need one integrated system.
There are three ways to mix Assembly and C:
- Using Assembly-defined functions into C
- Using C-defined functions into Assembly
- Using Assembly code in C
We’ll explore them all in this tutorial.
Factorial Function in X86_64 Assembly
Lately I’ve been reading the book Programming from the Ground Up by Jonathan Barlett. It teaches x86 assembly language programming from the very grounding blocks. Applying the concepts learnt in the book, I’ll show you how to write a factorial function in x86_64 Assembly.
Is It a Cat or a Dog? A Neural Network Application in OpenCV
Who is the good boy?
From time to time, a website named Kaggle hosts several competitions in the fields of Data Science and Computer Vision. One of those competitions was the Dogs vs. Cats challenge, where the objective was “to create an algorithm to distinguish dogs from cats”. Although this particular challenge already has been finished, I thought that it’d give me a pretty good material to a tutorial. Let’s learn how to solve this problem together using OpenCV!
Solving the Sliding Puzzle
Sliding puzzle is a game composed by 2n - 1 pieces dispersed along a board and a blank space. Those pieces are then shuffled and the objective is to rearrange them back to their original form, where the disposition of pieces is on ascending order, like shown below (go on, it’s interactive):
You can rearrange the pieces “moving” the blank space across the board. Since you can only move it in four directions, it’s a hell of a task to solve this game for a human, sometimes taking hours. Luckily, we dispose of some good algorithms to solve it, taking only few milliseconds even for the worst case. Let’s explore them in this tutorial! :)
Fingertip Detection in OpenCV
Hi! In this tutorial, we will learn how to detect fingertips using OpenCV. You ready? :D
Parallel Genetic Algorithm in C++ Using TBB
I’ve just finished my AI course, and the idea of evolutionary computing has appealed me incredibly. Basically, evolutionary computing is a set of algorithms inspired by Charles Darwin’s Theory of Evolution with intent of providing some intel on solving optimization problems. On this category, it’s included its most prominent example, the Genetic Algorithm.
But what is the Genetic Algorithm? It’s really pretty simple (and beautiful): Genetic Algorithm is a heuristic to search for the best solution of a optimization problem (such as the Knapsack problem, finding the best parameters of a neural network, and many others…), the ‘best’ here having direct analogy to the Theory of Evolution ‘survival of the fittest’.
(Python 2.7) Mesquita
Ahhh, and good and old (or not so old) statistics.
I create this software to help with my homework. In the end, just by doing it I was able to learn much more about statistical models. :)
Also, this software was my first attempt in create something with Python GUI, Tkinter. Wow, compared to Swing this one is like heaven!